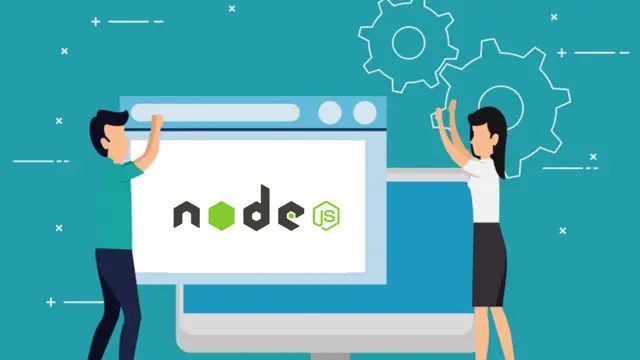
Node.js: Mastering Backend Development for Scalable Applications
Self-paced videos, Lifetime access, Study material, Certification prep, Technical support, Course Completion Certificate
Uplatz
Summary
- Reed Courses Certificate of Completion - Free
- Uplatz Certificate of Completion - Free
- Tutor is available to students
Add to basket or enquire
Overview
Uplatz offers this comprehensive course on Node.js: Mastering Backend Development for Scalable Applications. It is a self-paced course with video lectures. You will be awarded Course Completion Certificate at the end of the course.
Node.js is an open-source, cross-platform runtime environment built on Chrome's V8 JavaScript engine. It enables developers to run JavaScript on the server side, allowing for the creation of dynamic web applications, APIs, and other server-side functionalities.
How Node.js Works
Single-Threaded Event Loop Model:
- Node.js uses a single-threaded event loop architecture to handle multiple client requests efficiently.
- Incoming requests are placed into an event queue, and the event loop processes them asynchronously.
Non-Blocking I/O:
- Input/output operations, such as reading files or querying databases, are performed asynchronously using callbacks, Promises, or Async/Await.
- This ensures that other tasks are not blocked while waiting for operations to complete.
V8 JavaScript Engine:
- Node.js uses Google’s V8 engine, which compiles JavaScript directly into machine code, providing high performance.
Modules:
- Node.js has a modular design and uses a built-in module system (CommonJS) for code reuse.
- Additional functionality is available via npm packages.
Core Features of Node.js
Asynchronous and Event-Driven:
- All APIs are asynchronous and follow a non-blocking approach, making it highly efficient.
Fast Execution:
- Node.js is built on the V8 engine, which provides fast execution of JavaScript code.
Single Programming Language:
- Developers can use JavaScript for both frontend and backend, simplifying development.
Cross-Platform:
- Node.js applications can run on Windows, macOS, and Linux.
Built-in Modules:
- Includes modules for HTTP, file systems, streams, and more, reducing development time.
Scalability:
- Handles a large number of simultaneous connections efficiently using an event-driven architecture.
npm (Node Package Manager):
- Provides access to thousands of reusable libraries and tools for various functionalities.
Real-Time Applications:
- Ideal for real-time applications like chat applications, gaming servers, and live collaboration tools.
Benefits of Learning Node.js
High Demand:
- Node.js is widely used in the industry, creating opportunities for roles such as Backend Developer and Full Stack Developer.
Versatility:
- Enables the development of a wide range of applications, including web, mobile, and APIs.
Fast Learning Curve:
- For JavaScript developers, transitioning to Node.js is easy, as it uses the same language.
Community Support:
- A large and active community offers extensive resources, libraries, and tools.
Job Opportunities:
- Node.js is a critical skill for roles in modern web development, cloud computing, and API development.
Real-Time App Development:
- Simplifies building applications like chat apps, streaming platforms, and online collaboration tools.
Cost-Effective Development:
- Allows code sharing between frontend and backend, reducing development time and effort.
Scalability for Modern Apps:
- Suitable for applications requiring scalability, such as social media platforms or e-commerce sites.
Certificates
Reed Courses Certificate of Completion
Digital certificate - Included
Will be downloadable when all lectures have been completed.
Uplatz Certificate of Completion
Digital certificate - Included
Course Completion Certificate by Uplatz
Curriculum
Course media
Description
Node.js - Course Syllabus
Module 1. Introduction to Node.js
- Node.js Basics
- Overview of Node.js
- Initial concepts and advantages of using Node.js
Module 2. Core Concepts
- Core Modules and File Systems
- Introduction to Node.js core modules
- Working with the file system (examples included)
- Node Package Manager (NPM)
- NPM commands and examples
- Overview of the package.json file
- Yarn
- Introduction to Yarn
- Comparison between Yarn and NPM
Module 3. Building Blocks
- Simple HTTP Server with Node
- Create a basic HTTP server
- Add enhancements and features
- Routing Basics
- Simple routes, dynamic routes, and query parameters
Module 4. Asynchronous Programming
- Handling Asynchronous Code
- Using Callbacks, Promises, and Async/Await
Module 5. Modular Development
- Creating and Using Custom Modules
- Step-by-step examples
Module 6. Middleware and Debugging
- Middleware in Node.js
- Different middleware types, including Express examples
- Debugging and Error Handling
- Techniques for debugging and managing errors
Module 7. Development Tools
- Nodemon Tutorial
- Automating server restarts during development
Module 8. Express.js
- Introduction to Express
- Setting up and using Express.js
- Middleware in Express
- Exploring various middleware options
- Creating RESTful APIs with Express
- Developing RESTful APIs (examples included)
- Handling Query Parameters and Request Bodies
- Practical examples using Postman
Module 9. API Testing
- Introduction to Postman
- Features and usage for API testing
Module 10. NoSQL Databases (MongoDB)
- Understanding NoSQL Databases
- Basics of NoSQL and MongoDB
- Overview of MongoDB Features
- Introduction to Mongoose
- Overview and benefits
- Setting Up MongoDB
- Local setup and MongoDB Atlas configuration
- Installing Mongoose
- Setting up a basic project with Mongoose
- CRUD Operations in MongoDB
- Hands-on examples
Module 11. Advanced MongoDB and Mongoose
- MongoDB Virtuals, Getters, and Setters
- Middleware in Mongoose
- MongoDB Schema Relationships
- Aggregation Framework
- $match, $group, $sort, $project stages
- Mongoose Aggregation Queries
- Mongoose Schema Validation
- Built-in and custom validation techniques
- Handling Errors
- Validation and connection errors
- Creating and Using Indexes
- Examples and optimization tips
- Optimizing Mongoose Queries
- Transactions in MongoDB
- Using transactions effectively
- Role-Based Access Control (RBAC)
- Implementation with Mongoose
- Designing Data Models in MongoDB
- Integrating Mongoose with Express
- Creating RESTful APIs
Module 12. Deployment and Monitoring
- Deploying MongoDB Applications
- Monitoring and Maintenance Techniques
Module 13. EJS (Embedded JavaScript Templates)
- Getting Started with EJS
- Setting Up an EJS Project
- Passing Data to Templates
- Using Partials and Static Assets
- Conditional Rendering and Loops
- Error Handling in EJS
Module 14. Relational Databases (PostgreSQL)
- Introduction to PostgreSQL
- Basics and setup using psql and pgAdmin
- Basic SQL Commands
- PostgreSQL Data Types, Primary Keys, and Constraints
- Advanced SQL (SELECT, Joins, Views, Indexes)
- Transactions and Concurrency
- Functions, Procedures, and Triggers
- Working with JSON and Arrays
- Full-Text Search
Module 15. PostgreSQL with Node.js
- Integrating PostgreSQL with Node
- Backend Development with PostgreSQL and Express
- Relationships and Joins
- Using Knex.js for Query Building
Module 16. Security and Best Practices
- User Authentication with JWT
- Middleware and Validation (using Joi)
- Security Best Practices
Module 17. Advanced Development
- Migrations and Seeders
- Frontend Integration
- Using React.js, Fetch, and Axios
Module 18. Final Touches
- Interview Questions and Answers
- Key concepts and code snippets for interview preparation
Who is this course for?
Everyone
Requirements
Passion and determination to achieve your goals!
Career path
- Node.js Developer
- Backend Developer
- Full Stack Developer
- API Developer
- JavaScript Developer
- Express.js Developer
- MongoDB Developer
- PostgreSQL Developer
- Web Developer
- Software Engineer
- Technical Lead
- Solution Architect
- Cloud Developer
- DevOps Engineer
- Middleware Engineer
- Database Administrator
- API Integration Specialist
- RESTful API Developer
- Data Engineer
- Application Support Engineer
Questions and answers
Currently there are no Q&As for this course. Be the first to ask a question.
Reviews
Currently there are no reviews for this course. Be the first to leave a review.
Legal information
This course is advertised on Reed.co.uk by the Course Provider, whose terms and conditions apply. Purchases are made directly from the Course Provider, and as such, content and materials are supplied by the Course Provider directly. Reed is acting as agent and not reseller in relation to this course. Reed's only responsibility is to facilitate your payment for the course. It is your responsibility to review and agree to the Course Provider's terms and conditions and satisfy yourself as to the suitability of the course you intend to purchase. Reed will not have any responsibility for the content of the course and/or associated materials.