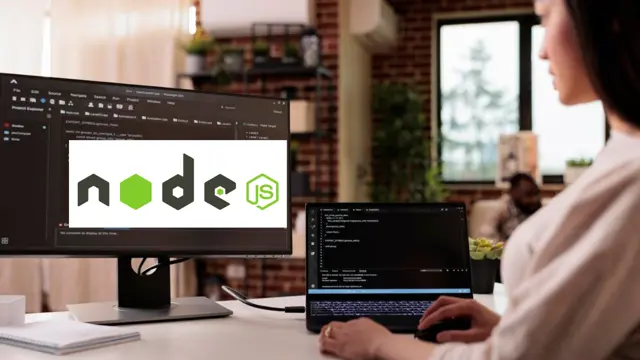
Node.js Programming Course
Self-paced videos, Lifetime access, Study material, Certification prep, Technical support, Course Completion Certificate
Uplatz
Summary
- Reed courses certificate of completion - Free
- Uplatz Certificate of Completion - Free
Add to basket or enquire
Overview
Uplatz offers this comprehensive course on Node.js. It is a self-paced course with video lectures. You will be awarded Course Completion Certificate at the end of the course.
Node.js is an open-source, cross-platform, back-end JavaScript runtime environment that executes JavaScript code outside of a web browser. It's built on the powerful Chrome's V8 JavaScript engine, renowned for its performance and efficiency. Node.js is used for building scalable and high-performance network applications such as web servers, real-time chat applications, APIs, and microservices.
Key Characteristics
- Asynchronous and Event-Driven: Node.js operates on an event loop, which allows it to handle multiple requests concurrently without blocking the execution of other tasks. This non-blocking I/O model makes it highly efficient for applications dealing with many simultaneous connections.
- Single-Threaded but Highly Scalable: While Node.js utilizes a single thread for event processing, it can handle a large number of concurrent connections due to its asynchronous nature. This makes it a suitable choice for building scalable applications.
- Cross-Platform Compatibility: Node.js can run on various operating systems, including Windows, macOS, and Linux, providing flexibility in development and deployment.
Core Features and Functionality
- Modules: Node.js has a modular architecture, allowing developers to organize code into reusable units. It provides a rich set of built-in modules for common tasks like file system access, network communication, and data streams.
- NPM (Node Package Manager): NPM is the default package manager for Node.js and boasts the largest ecosystem of open-source libraries in the world. It simplifies the process of incorporating external modules into your projects, fostering code reuse and community collaboration.
- File System I/O: Node.js provides a comprehensive API for interacting with the file system, enabling operations such as reading, writing, and deleting files and directories.
- Networking: Node.js excels in network programming, offering powerful modules for creating network applications. It supports various protocols, including TCP, HTTP, and UDP, facilitating the development of web servers, client-server applications, and real-time communication systems.
- Streams: Node.js provides streams, which are objects that allow for efficient handling of large amounts of data. Streams enable processing data in chunks, reducing memory consumption and improving performance, particularly when dealing with files or network requests.
- Child Processes: Node.js allows you to spawn child processes to execute external commands or run other Node.js scripts, enabling parallel processing and offloading computationally intensive tasks.
- Cluster Module: For utilizing multiple CPU cores and enhancing application performance, Node.js offers the Cluster module. It facilitates the creation of child processes that share server ports, effectively distributing the workload.
Benefits of Learning Node.js
- Full-Stack Development: Leverage your JavaScript skills for both front-end and back-end development, streamlining the development process and reducing context switching.
- High Performance: Build fast and scalable network applications due to Node.js's non-blocking, event-driven architecture.
- Increased Employability: Node.js is in high demand across various industries, making it a valuable skill for developers seeking career opportunities.
- Microservices Architecture: Node.js is well-suited for building microservices, which are small, independent, and loosely coupled services that can be developed, deployed, and scaled individually.
- Fast Development: Node.js promotes rapid prototyping and development due to its lightweight nature, extensive module ecosystem, and JavaScript's ease of use.
- Real-time Applications: Node.js is ideal for building real-time applications like chat applications, online games, and collaboration tools.
Use Cases:
- Web Servers and APIs: Node.js is widely used for developing RESTful APIs and web servers due to its efficiency in handling HTTP requests and JSON data.
- Real-time Applications: Its event-driven architecture makes it perfect for chat applications, online games, and collaborative tools.
- Microservices: Node.js is a popular choice for building microservices due to its lightweight nature and ability to handle independent deployments.
- Command-line Tools: Node.js can be used to create command-line tools for automating tasks and managing system operations.
- Desktop Applications: Frameworks like Electron allow you to build cross-platform desktop applications using Node.js and web technologies.
Certificates
Reed courses certificate of completion
Digital certificate - Included
Will be downloadable when all lectures have been completed
Uplatz Certificate of Completion
Digital certificate - Included
Course Completion Certificate by Uplatz
Curriculum
Course media
Description
Node.js - Course Syllabus
I. Introduction & Foundations
- Getting Started with Node.js
- Installing and Running Node.js
- Hello World in the REPL
- Hello World command line
- Hello World HTTP server
- Hello World basic routing
- Hello World with Express
- Debugging Your NodeJS Application
- Deploying your application online
- Core Modules
- The global object
- TLS Socket: server and client
- How to get a basic HTTPS web server up and running!
- File System
- The FS Module
- Reading Files
- Reading Directories
- Streams
II. Node.js Ecosystem & Development
- Npm (Node Package Manager)
- Setting up a package configuration
- Installing packages
- Uninstalling packages
- Listing currently installed packages
- Removing extraneous packages
- Running scripts
- Basic semantic versioning
- Publishing a package
- CommonJS Modules
- Directories as Modules
- npm Packages
- Managing Dependencies
- npm scripts
- Node.js Event Loop & Asynchronous Programming
- The Node.js process
- Asynchronous Javascript
- Asynchronous Control Flow with Callbacks
- Promises
- Async/Await
- The EventEmitter API
- Third-Party Async packages
III. Building Web Applications & APIs
- Building Servers & HTTP
- Creating servers with HTTP
- The Http server class
- Parsing requests
- HTTP streaming
- Building APIs using modules, events and packages
- ExpressJS Framework
- Introduction to ExpressJS
- Routing
- Responding
- Configuration
- Views
- Middlewares
- Receiving Data
- Error Handling
- RESTful APIs
- REST
- Authentication
- Stateful vs. Stateless Authentication
- OAuth2
- Passport
- JWT – JSON Web Tokens
- Working With Data
IV. Deployment & Best Practices
- Hosting & Deployment
- Why do we need hosts
- Forever
- PM2
- Node on Windows
- Node as a Windows Service
- IISNode
- Hosting Complex Node Architectures with Docker
- What is Docker
- Docker CLI
- Docker File system & Volumes
- Docker Files
- Containers Communication
- Docker Compose
- Best Practices
- NPM Best Practices
- Node.js API design
- Error Handling
- Debugging
Who is this course for?
- Frontend developers looking to expand into full-stack development with Node.js
- Beginners in backend web development who want to learn server-side JavaScript
- JavaScript developers who want to work on backend services, APIs, and servers
- Software developers aiming to build web applications using Node.js and Express
- Developers transitioning from other backend languages (like PHP, Python, or Ruby) to Node.js
- Programmers who want to understand how to create and deploy RESTful APIs
- Full-stack developers who need a deeper understanding of the Node.js ecosystem
- Developers learning to work with file systems, streams, and core Node.js modules
- Application developers who need to manage asynchronous code using callbacks, promises, and async/await
- Engineers working on authentication systems using JWT, OAuth2, and Passport
- Professionals involved in API development, microservices, or scalable server-side applications
- Developers seeking experience with deployment tools like PM2, Docker, and process managers
- IT professionals responsible for deploying and managing Node.js applications in production
- Teams using Docker and wanting to integrate Node.js into containerized environments
- Programmers interested in learning best practices for debugging, error handling, and API design
- Students studying web development, backend systems, or JavaScript frameworksCoding boo
- tcamp participants learning full-stack development
- Freelancers and independent developers building backend services or SaaS platforms
- Career changers entering software development with a focus on backend technologies
- Technical trainers or educators creating material on modern web backend development
Requirements
Passion to learn and succeed!
Career path
- Node.js Programmer
- JavaScript Developer
- Software Developer
- IT Programmer
- Full Stack Web Developer
- Front End Web Developer
- Data and Apps Engineer
Questions and answers
Currently there are no Q&As for this course. Be the first to ask a question.
Reviews
Currently there are no reviews for this course. Be the first to leave a review.
Legal information
This course is advertised on Reed.co.uk by the Course Provider, whose terms and conditions apply. Purchases are made directly from the Course Provider, and as such, content and materials are supplied by the Course Provider directly. Reed is acting as agent and not reseller in relation to this course. Reed's only responsibility is to facilitate your payment for the course. It is your responsibility to review and agree to the Course Provider's terms and conditions and satisfy yourself as to the suitability of the course you intend to purchase. Reed will not have any responsibility for the content of the course and/or associated materials.