- Reed courses certificate of completion - Free
- Uplatz Certificate of Completion - Free
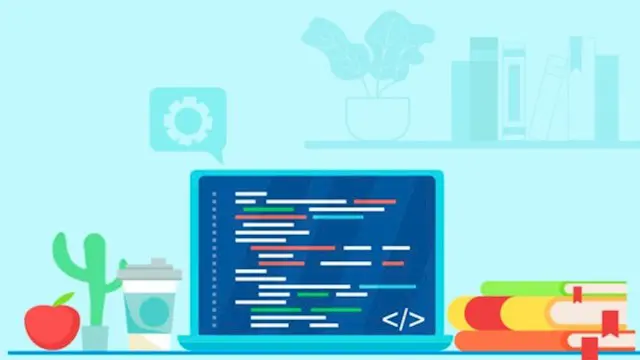
Bundle Course - Java (Core Java, JSP, Java Servlets)
Self-paced videos, Lifetime access, Study material, Certification prep, Technical support, Course Completion Certificate
Summary
Overview
Uplatz offers this comprehensive bundle course on Java consisting of a combo of video courses on all topics that are associated with Java (Core Java, JSP, Java Servlets). You will be awarded Course Completion Certificate at the end of the course.
Uplatz provides this comprehensive training on Java with a combo of courses included together in this bundle course. This is self-paced training in the form of pre-recorded videos.
The courses included in the Java bundle course are:
- Java Programming
- Java Server Pages (JSP)
- Java Servlets
Java is one of the most used and most popular programming languages in the world of IT development. Given its properties of platform independence, object-oriented approach, reusable code and dynamic classes, Java has emerged to be the most preferred language for programmers. It is quite easy to learn and implement, thus making it highly favorable in the programming community. Its popularity also stems from the fact that it was built keeping security in mind, and enables programmers to write code with fewer bugs, in lesser time.
As Java is secure and multi-threaded, it is perfect for Banking and transaction management services. E-commerce shops and billing software have their logic written in frameworks based on Core Java. Mobile OS like Android uses Java APIs. Stock market algorithms are based out of Java. Java along with other frameworks like Spring makes for a robust combination to sort implementation dependencies and write server-side applications in Finance and IT domains.
Java is fast, reliable and secure. From desktop to web applications, scientific supercomputers to gaming consoles, cell phones to the Internet, Java is used in every nook and corner. Java is a very successful language and it has gained huge popularity.
Java Server Pages (JSP) is a server-side programming technology that enables the creation of dynamic, platform-independent method for building Web-based applications. JSP have access to the entire family of Java APIs, including the JDBC API to access enterprise databases. This tutorial will teach you how to use Java Server Pages to develop your web applications in simple and easy steps.
Applications of JSP
As mentioned before, JSP is one of the most widely used language over the web. I'm going to list few of them here:
JSP vs. Active Server Pages (ASP)
The advantages of JSP are twofold. First, the dynamic part is written in Java, not Visual Basic or other MS specific language, so it is more powerful and easier to use. Second, it is portable to other operating systems and non-Microsoft Web servers.
JSP vs. Pure Servlets
It is more convenient to write (and to modify!) regular HTML than to have plenty of println statements that generate the HTML.
JSP vs. JavaScript
JavaScript can generate HTML dynamically on the client but can hardly interact with the web server to perform complex tasks like database access and image processing etc.
JSP vs. Static HTML
Regular HTML, of course, cannot contain dynamic information.
Java Servlet technology is used to create a web application (resides at server side and generates a dynamic web page).
Servlet technology is robust and scalable because of java language. Before Servlet, CGI (Common Gateway Interface) scripting language was common as a server-side programming language. However, there were many disadvantages to this technology. We have discussed these disadvantages below.
There are many interfaces and classes in the Servlet API such as Servlet, GenericServlet, HttpServlet, ServletRequest, ServletResponse, etc.
Properties of Servlets:
- Servlets work on the server-side.
- Servlets are capable of handling complex requests obtained from web server.
Web application development can be made easier by using Java servlets. In the Java Servlet topic viz. Java EE: Programming Servlets, you will begin with a complete overview of servlet architecture and lifecycle. First, you'll see the configuration of a Tomcat webserver in Eclipse and you'll learn how to read the request and response headers. Next, you'll learn how filters are applied to servlets and see many details about tracking session data, web annotations, and globalizing servlets. Finally, you'll go over asynchronous programming in servlets, debugging, packing, and deployment of servlets. By the end of this course, you will have a much more complete understanding of how web development using Java servlets works. Software required: Tomcat and Eclipse.
Java servlets are the first step to understanding web programming using Java, and this course will show you the basics of servlet architecture and applying filters to more complex issues like debugging and deployment.
Certificates
Reed courses certificate of completion
Digital certificate - Included
Will be downloadable when all lectures have been completed
Uplatz Certificate of Completion
Digital certificate - Included
Course Completion Certificate by Uplatz
Curriculum
Course media
Description
Java Programming - course curriculum
Java Overview
• How the Java Environment Works
• HelloWorld Program
• Launch Single-File Source Code
• jShell REPL
• Comments and Terminators
• Identifiers
Syntax and Types
• Java Variables
• var declarations
• Java Types
• Basic Java Types
• Reference types
• Conditional Expressions
• Logical operators
• If statement
• While Statement
• Do Statement
• For statement
• Special flow of control operators
• Switch statement
• Numerical Operators
• Casting
• Strings
Classes and Objects
• What is an Object?
• Objects and Encapsulation
• What is a Class?
• Class Object Relationship
Working with Methods
• Method Definitions
• Method Arguments & Return Types
• Overloading Methods
Class Inheritance
- Inheritance in Java
- Implementing Inheritance
- Rule for overriding methods
- Rules for Polymorphic variables
- Casting and Inheritance
- The super variable
- Constructors and Inheritance
Abstract Classes, Class Side Behavior and Final
- Abstract Classes
- Abstract Classes in Java
- Defining an Abstract Class
- Extending an Abstract Class
- Using Concrete Subclasses
- Class Side Information
- Class Side Data
- Class Side Behaviour
- Final Keyword
Java Interfaces and Enumerations
- What is an Interface in Java?
- Basic Interface Definitions
- Implementing an Interface
- Interface
- Using an Interface in a Contract
- Inheritance by Interfaces and Types
Packages
- Packages
- Class-Package Relationship
- Declaring Packages
- Packaging the Person class
- Role of the Classpath
- JAR Files
- Encapsulation and Packages
- Class modifier
- Constructor Modifier
- Variable Modifier
- Method Modifiers
- Package Summary
Java 9+ Modules
- Introduction the JPMS
- Java Platform Module System
- Why we need modules
Arrays
- What is a Java Array?
- Creating arrays of Objects
- Accessing Array Elements
- Main method args array
- Ragged Arrays in Java
- Working with Ragged Arrays
- Implications of Inheritance for Arrays
- Integer Array Example
Java Generics
- Generics and Basic Types
- Generics and Their Types
- Adding Generics to your classes
- A simple user defined Generic class (the Bag class)
- Type Equality
- Generic Collection Assignment
- Generics and Inheritance
Collections Classes
- Collections API
- ArrayList
- Interfaces v Concrete Classes
- HashMap
- Iteration and Enumeration
- Queues
- Generics and Collections
- For Loops
- Boxing and Unboxing
- Raw Collections
Java 9+ Immutable Collections
Error and Exception Handling
Nested / Inner Classes
- Four types of Nested / Inner Class
- Properties of Member level inner classes
- Properties of Method Inner classes
- Anonymous Method Inner classes
- Java 11 Nest-based Access
Java Functions
Java Optional Type
- Null considered Harmful
- Java Optional Type
- Optional Variables
- Creating Optional values
- Method Summary
Java 9 Streams
- Streams
- Streams from Collections
- Terminal / Non Terminal Operators
- Creating a Stream
- Map Operation
- Collectors
- Filter operation
- Sorted operation
- ForEach
- Pipelining Operations
- Parallel Streams
- Not just collections
Files, Paths and IO Streams
- Introducing NIO.2
- Paths class and Path Interface
- The Files class
- File Attributes
- The File Watcher Service
- IO Streams
- Scanners
------
Java Server Pages (JSP) - course curriculum
1. Web Applications
Server-Side Programming
Web Protocols and Web Applications
Role of Web Servers
Java Servlets
Using Tomcat Web server
Structure of a Java Servlet
2. Servlets Architecture
Servlets Architecture
Servlet and HttpServlet
Request and Response
Reading Request Parameters
Producing an HTML Response
Redirecting the Web Server
Deployment Descriptors
Servlets Life Cycle
Relationship to the Container
3. Interactive Web Applications
Building an HTML Interface
HTML Forms
Handling Form Input
Application Architecture
Single-Servlet Model
Multiple-Servlet Model
Routing Servlet Model
Template Parsers
4. Session Management
Managing Client State
Sessions
Session Implementations
HttpSession
Session Attributes
Session Events
Invalidating Sessions
5. Configuration and Context
The Need for Configuration
Initialization Parameters
Properties Files
JNDI and the Component Environment
JDBC Data Sources
Working with XML Data
6. Filters
Servlet Filters
Uses for Filters
Building a Filter
Filter Configuration and Context
Filter Chains
Deploying Filters
7. Database and SQL Fundamentals
Relational Databases and SQL
SQL Versions and Code Portability
Database, Schema, Tables, Columns and Rows
DDL - Creating and Managing Database Objects
DML - Retrieving and Managing Data
Sequences
Stored Procedures
Result Sets and Cursors
Using SQL Terminals
8. JDBC Fundamentals
What is the JDBC API?
JDBC Drivers
Making a Connection
Creating and Executing a Statement
Retrieving Values from a ResultSet
SQL and Java Datatypes
SQL NULL Versus Java null
Creating and Updating Tables
Handling SQL Exceptions and Proper Cleanup
Handling SQLWarning
9. Advanced JDBC
SQL Escape Syntax
Using Prepared Statements
Using Callable Statements
Scrollable Result Sets
Updatable Result Sets
Transactions
Commits, Rollbacks, and Savepoints
Batch Processing
Alternatives to JDBC
10. Introduction to Row Sets
Row Sets in GUI and J2EE programming
Advantages of RowSets
RowSet Specializations
Using CachedRowSets
11. JSP Architecture
JSP Containers
Servlet Architecture
Page Translation
Types of JSP Content
Directives
Content Type
Buffering
Scripting Elements
JSP Expressions
Standard Actions
Custom Actions and JSTL
Objects and Scopes
Implicit Objects
JSP Lifecycle
12. Scripting Elements
Translation of Template Content
Scriptlets
Expressions
Declarations
Dos and Don'ts
Implicit Objects for Scriptlets
The request Object
The response Object
The out Object
13. Interactive JSP Applications
HTML Forms
Reading CGI Parameters
JSPs and Java Classes
Error Handling
Session Management
The Session API
Cookies and JSP
14. Using JavaBeans
Separating Presentation and Business Logic
JSP Actions
JavaBeans
Working with Properties
and
Using Form Parameters with Beans
Objects and Scopes
Working with Vectors
15. The Expression Language and the JSTL
Going Scriptless
The JSP Expression Language
EL Syntax
Type Coercio
Error Handling
Implicit Objects for EL
The JSP Standard Tag Library
Role of JSTL
The Core Actions
Using Beans with JSTL
The Formatting Actions
Scripts vs. EL/JSTL
16. Advanced JSP Features
Web Components
Forwarding
Inclusion
Passing Parameters
Custom Tag Libraries
Tag Library Architecture
Implementing in Java or JSP
Threads
Strategies for Thread Safety
XML and JSP
17. JSP for Web Services
--------
Java Servlets - course curriculum
- Introduction to World Wide Web
- Understanding static and Dynamic webpages
- How to create a HelloWorld application using servlets
- Servlet interface, Generic and Http Servlet interfaces
- Annotation and XML based configuration in Servlets
- Difference between Get & Post
- How Servlet works (Life cycle)
- Load On Start-up Configuration
- Request Scope in Servlets
- Request Dispatcher interface
- Inter-servlet communication using Response send.Redirect
- Servlet Config
- Servlet Context
- Session Tracking using cookies
- Hidden Form Fields
- URL Rewriting
- Http Session
- Filters in servlets
- Filter Config
- Database connection in servlets
- CRUD operations using servlets to MySQL database
- Events and Listeners in servlets
- Servlet Context Listener
- Http Session Listener
Who is this course for?
Everyone
Requirements
Passion and determination to achieve your goals!
Career path
- Java Developer
- Web Developer
- Application Developer
- Full Stack Software Engineer
- JSP and Java Servlets Developer
- Java Middleware Software Developer
- Java J2EE Developer
- Engineering Manager
- Solutions Architect
- Technical Architect
- Java Developer - Microservices, Kubernetes
- Lead Engineer
- Front-end Web Developer / Programmer
- Java, JSP, Servlet Programmer
- Mobile App Developer
- Technical Lead
Questions and answers
Am able to search for job in the field of database administrator at the completion of this course? Please an honest reply will be appreciated. Thanks
Answer:Hi Timi Yes, you'll be able to apply for DBA and PL-SQL Developer roles after completion of the course. We will also share with you a 211-page handbook on SQL which will really help you practice the skills you acquire during the course. Our suggestion is you take up our instructor-led online training course rather than a self-paced course since that you help you master SQL and DBA skills in great depth, ideal for preparing for a job interview. Regards Team Uplatz
This was helpful.Do you get a certificate, once you have completed the course, for your CV?
Answer:Yes, we will provide the Course Completion Certificate to you. Regards Team Uplatz
This was helpful.Hi, what is the completion time frame with this course? What happens if I was to fail the course? Could it be retaken? Kind regards Lynnette
Answer:Hi Lynnette The course duration is of 10 hours. This is self-paced course with pre-recorded videos that you can play online. There is no exam, so there is no option to fail at all! The purpose of this course is basically to equip you with knowledge of SQL (Structured Query Language) so that you can apply this skill in your job as well as to help you in a job interview for database programmer etc. Regards Indu Uplatz
This was helpful.
Reviews
Currently there are no reviews for this course. Be the first to leave a review.
Provider
Uplatz is leading global provider of IT & Technology training.
We have a strong network of qualified and experienced tutors. Uplatz provides training on cutting-edge technologies such as Data Science, Machine Learning, AWS, Microsoft Azure, Google Cloud, IBM Cloud, Data Engineering, Python, R, Java, SAP, Oracle, SAS, Salesforce, Web Development Stack, JavaScript, ReactJS, AngularJS, NodeJS, JSP & Java Servlets, MongoDB, BI Tools such as Tableau, Spotfire, Power BI, DW & ETL tools such as Informatica, IBM DataStage, Talend, DevOps, Project Management, Software Testing, and many more.
We provide training courses in both online formats - 1) live tutor-led, 2) self-paced videos
We feel proud to say that we are making learning affordable by keeping prices of all our courses very low. Our course prices are listed at almost 90% discounted rate from average market price.
Our Vision
- To become a global leader in the learning sector by providing training on job-oriented technologies
Our Mission
- To provide high-quality training on industry-demanded technologies
- To make learning affordable for the masses by keeping our prices extremely low
- To help our talented students get a high-paying job in the market
Will I get a Certificate of Course Completion?
Course Completion Certificate is awarded by Uplatz
What are your top courses?
- Data Science
- Machine Learning
- Cloud Computing - AWS, Azure, Google Cloud, IBM Cloud, and more
- Data Engineering
- SAP modules such as S/4HANA Finance, EWM, TRM, FICO, BPC, HCM, WM, MM, PP, PM, QM, SD, TRM, SuccessFactors, UI5 and Fiori, S/4HANA Logistics, TM, etc.
- Oracle
- SAS
- Salesforce
- BI Tools - Tableau, Power BI, Spotfire, MicroStrategy, etc.
- DW & ETL Tools - Informatica, Talend, IBM DataStage, etc.
- Project Management & DevOps
- Software Testing
- Digital Marketing & SEO
Will I be provided study material, tutor notes and practice assignments?
Uplatz provides tutor notes, practice assignments, practice sessions, and a lot of useful study material for free. This will help you in job interviews and certification exams.
In case of tutor-led online training, will I have access to the recorded sessions?
Yes. All live classes get recorded and life-time access on the recordings is provided.
Course Search and Enrollment
Simply search the course of your choice! We have a portfolio of more than 1000 courses with the premium ones highlighted clearly. Just search for the course that you want to take or simply use our online course finding tool to help you choose the best courses as per your needs and market demand.
Uplatz differentiates itself by providing extremely affordable learning to all and that too in the comfort of their homes.
Just contact us for a customized quote, your preferable timings, your affordability, and we'll work out the best course for you that will provide you not only a great return on investment but also to help you get a job with a premium salary. We'll also get you prepared for the certification exams.
Legal information
This course is advertised on Reed.co.uk by the Course Provider, whose terms and conditions apply. Purchases are made directly from the Course Provider, and as such, content and materials are supplied by the Course Provider directly. Reed is acting as agent and not reseller in relation to this course. Reed's only responsibility is to facilitate your payment for the course. It is your responsibility to review and agree to the Course Provider's terms and conditions and satisfy yourself as to the suitability of the course you intend to purchase. Reed will not have any responsibility for the content of the course and/or associated materials.
FAQs
Interest free credit agreements provided by Zopa Bank Limited trading as DivideBuy are not regulated by the Financial Conduct Authority and do not fall under the jurisdiction of the Financial Ombudsman Service. Zopa Bank Limited trading as DivideBuy is authorised by the Prudential Regulation Authority and regulated by the Financial Conduct Authority and the Prudential Regulation Authority, and entered on the Financial Services Register (800542). Zopa Bank Limited (10627575) is incorporated in England & Wales and has its registered office at: 1st Floor, Cottons Centre, Tooley Street, London, SE1 2QG. VAT Number 281765280. DivideBuy's trading address is First Floor, Brunswick Court, Brunswick Street, Newcastle-under-Lyme, ST5 1HH. © Zopa Bank Limited 2025. All rights reserved.