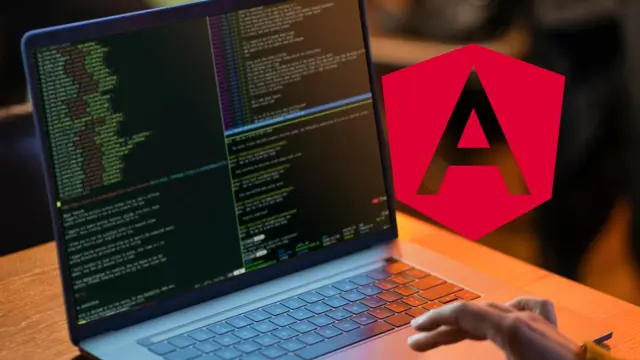
Angular: Full-Stack Application Development
Self-paced videos, Lifetime access, Study material, Certification prep, Technical support, Course Completion Certificate
Summary
- Reed Courses Certificate of Completion - Free
- Uplatz Certificate of Completion - Free
Add to basket or enquire
Overview
Uplatz offers this comprehensive course on Angular: Full-Stack Application Development. It is a self-paced course with video lectures. You will be awarded Course Completion Certificate at the end of the course.
What is Angular?
Angular is a popular open-source web application framework developed and maintained by Google. It is used for building dynamic, single-page applications (SPAs) and progressive web apps (PWAs). Angular is a TypeScript-based framework that provides a structured way to develop web applications by offering a comprehensive suite of tools and features.
Angular is a powerful framework for modern web development, offering a robust set of tools and features to build dynamic, scalable, and maintainable applications. Its component-based architecture, TypeScript support, and comprehensive ecosystem make it a popular choice for developers and organizations worldwide. Whether you're building a small project or a large enterprise application, Angular provides the tools and structure needed to succeed.
How Angular Works
Angular works by extending HTML with additional attributes and using a component-based architecture. Here's a high-level overview of how Angular works:
Components: Angular applications are built using components. Each component consists of:
A TypeScript class that defines the behavior (logic).
An HTML template that defines the view (UI).
CSS styles for styling the component.
Modules: Angular apps are modular, and Angular uses NgModules to organize the application into cohesive blocks of functionality. Every Angular app has at least one root module (AppModule).
Templates: Angular uses HTML templates to define the UI. These templates can include Angular-specific syntax like data binding, directives, and pipes.
Data Binding: Angular provides a powerful data binding mechanism that synchronizes the data between the component and the view. There are four forms of data binding:
Interpolation ({{ }})
Property binding ([ ])
Event binding (( ))
Two-way binding ([( )])
Dependency Injection: Angular has a built-in dependency injection system that makes it easier to develop and test applications by providing dependencies to components and services.
Routing: Angular's router enables navigation between different views or components based on the URL. It allows for lazy loading of modules, which improves performance.
Services: Services are used to encapsulate business logic, data access, or shared functionality. They can be injected into components.
Directives: Angular provides built-in directives (e.g. ngIf, ngFor) to manipulate the DOM and add dynamic behavior to the application.
Pipes: Pipes are used to transform data in the template, such as formatting dates, currency, or filtering lists.
Change Detection: Angular uses a change detection mechanism to automatically update the view whenever the underlying data changes.
Key Features of Angular
Two-Way Data Binding: Simplifies the synchronization between the model and the view.
Component-Based Architecture: Encourages reusability and modularity.
TypeScript Support: Provides strong typing, better tooling, and improved code quality.
Dependency Injection: Makes applications more modular and testable.
RxJS Integration: Simplifies handling asynchronous operations and event-based programming.
Angular CLI: A command-line tool for scaffolding, building, and testing Angular applications.
Cross-Platform Development: Supports building web, mobile (via Ionic), and desktop (via Electron) applications.
Powerful Templating: Combines HTML with Angular directives for dynamic content.
Built-in Routing: Simplifies navigation and lazy loading.
Comprehensive Tooling: Includes tools for testing, debugging, and optimizing applications.
Benefits of Angular
Enhanced Productivity: Angular's CLI, component-based architecture, and reusable code reduce development time.
Improved Performance: Features like lazy loading, Ahead-of-Time (AOT) compilation, and tree-shaking optimize performance.
Scalability: Angular is designed for building large-scale applications with maintainable code.
Cross-Platform Support: Enables developers to build applications for web, mobile, and desktop using a single framework.
Strong Community and Ecosystem: Backed by Google and a large community, Angular has extensive documentation, third-party libraries, and tools.
TypeScript Advantages: Strong typing, better IDE support, and improved code maintainability.
Testing Support: Built-in support for unit testing and end-to-end testing.
Consistency: Angular provides a consistent structure and best practices, making it easier for teams to collaborate.
Security: Built-in protections against common web vulnerabilities like XSS and CSRF.
Certificates
Reed Courses Certificate of Completion
Digital certificate - Included
Will be downloadable when all lectures have been completed.
Uplatz Certificate of Completion
Digital certificate - Included
Course Completion Certificate by Uplatz
Curriculum
Course media
Description
Angular - Course Syllabus
Module 1: Angular Foundations
- 1. What is Angular?
- Introduction to Angular and its benefits.
- Why learn Angular for modern web development.
- 2. Angular 19 Setup
- Installing Angular CLI.
- Creating a new Angular application.
- Troubleshooting common setup issues.
- 3. First Changes in Angular App
- Basic modifications to app.component.html and app.component.ts.
- Understanding the initial application structure.
- 4. Angular 19 File and Folder Structure
- Detailed explanation of the Angular project architecture.
- Understanding the purpose of key files and folders.
- 5. Interpolation in Angular
- Displaying data in templates using interpolation.
- Practical examples of data binding.
- 6. Angular CLI
- Essential Angular CLI commands for development.
- Generating components, services, and modules.
Module 2: Core Angular Concepts
- 7. Angular Components
- Understanding the role of components in Angular.
- Component lifecycle and data flow.
- 8. Creating a Custom Component
- Manual creation of reusable components.
- Component interaction and best practices.
- 9. Function Calls on Button Click in Angular
- Handling user interactions with event binding.
- Implementing event handlers and methods.
- 10. Defining Data Types in Angular
- Using TypeScript data types for robust applications.
- Type safety and best practices.
- 11. Build a Counter App in Angular
- Hands-on project: building a simple counter application.
- Applying core Angular concepts.
- 12. Important Events in Angular
- Exploring common DOM events in Angular.
- Practical examples of event handling.
- 13. Getting and Setting Input Field Values in Angular
- Two-way data binding and form input handling.
- Practical input field manipulation.
- 14. Styling in Angular
- Component-specific styling and global styles.
- Using CSS, SCSS, and other styling techniques.
- 15. Conditional Rendering with if-else and Toggle Functionality
- Using *ngIf for conditional rendering.
- Implementing toggle functionality.
- 16. Control Flow with else if
- Expanding conditional logic with *ngIf and else if.
- 17. Using switch-case in Angular
- Implementing switch-case logic in templates.
- 18. Using for Loop in Angular
- Iterating over data with *ngFor.
- 19. Angular Signals
- Introduction to Angular Signals for reactive state management.
- 20. Data Types with Signals
- Using various data types with Signals.
- 21. Computed Signals
- Utilizing computed signals for derived values.
- 22. Angular Effects
- Understanding and using Angular Effects.
- 23. @for Loop and Contextual Variables
- Exploration of the @for loop and contextual variables.
- 24. Two-Way Binding
- Implementing two-way data binding with ngModel.
- 25. To-Do List
- Hands-on project: building a to-do list application.
- 26. Angular Dynamic Styling
- Applying dynamic styles based on component state.
- 27. Directives in Angular
- Introduction to structural and attribute directives.
- 28. ngFor Directive
- Advanced usage of the ngFor directive.
- 29. ngIf Directive
- Using the ngIf directive and else blocks.
- 30. ngSwitch Directive
- Implementing switch-case logic with ngSwitch.
Module 3: Routing, Forms, and Data Management
- 31. Basic Routing in Angular
- Setting up routes and navigation.
- Creating single-page applications.
- 32. Adding a Header with Routing
- Implementing a navigation header with routing.
- 33. Creating a 404 Page
- Handling invalid routes with a 404 page.
- 34. Passing Data Between Pages
- Passing data using route parameters and query parameters.
- 35. Dynamic Routing
- Implementing dynamic routes with parameters.
- 36. Forms in Angular
- Introduction to template-driven and reactive forms.
- 37. Basic Reactive Forms
- Creating forms with reactive form APIs.
- 38. Reactive Forms with Form Grouping
- Organizing forms with form groups.
- 39. Reactive Form Validation
- Implementing form validation with reactive forms.
- 40. Template-Driven Forms
- Creating forms with template-driven approach.
- 41. Angular Template-Driven Forms Validation
- Validation of Template driven forms.
- 42. Passing Data from Parent to Child
- Using @Input to pass data between components.
- 43. Reusable Component Example
- Building reusable components.
- 44. Passing data from a Child Component to a Parent Component
- Using @Output and event emitters.
- 45. Angular Pipes
- Transforming data with built-in pipes.
- 46. Creating Custom Pipes in Angular
- Building custom data transformation pipes.
- 47. Angular Lifecycle Hooks Tutorial
- Understanding component lifecycle hooks.
- 48. Angular Services
- Creating and using Angular services for data sharing.
- 49. Calling API with Services in Angular
- Making HTTP requests with Angular services.
- 50. Using Data Types for API Results
- Typing API responses.
- 51. Post API Tutorial
- Implementing POST requests.
- 52. Delete API Tutorial
- Implementing DELETE requests.
- 53. Populate Data in Input Fields with API
- Populating forms with API data.
- 54. Update Data with PUT API Method
- Implementing PUT requests.
- 55. Route Lazy Loading
- Improving performance with lazy loading.
Module 4: Advanced Angular and Deployment
- 56. Build and Local Deploy
- Building and deploying Angular applications locally.
- 57. Deploying an Angular App on Vercel
- Deploying Angular applications to Vercel.
- 58. Setup Tailwind CSS in Angular
- Integrating Tailwind CSS for styling.
- 59. Introduction to RxJS in Angular
- Understanding RxJS observables and operators.
- 60. RxJS Observables vs Angular Signals
- Comparison of Signals and Observables.
- 61. Angular Dependency Injection (DI)
- Understanding and using dependency injection.
- 62. Optimizing Performance in Angular
- Techniques for optimizing Angular application performance.
Who is this course for?
Everyone
Requirements
Passion and zeal to accomplish something big!
Career path
- Angular Developer
- Frontend Developer
- Full-Stack Developer
- UI/UX Developer
- Web Developer
- Software Engineer
- JavaScript Developer
- TypeScript Developer
- Mobile App Developer (using Ionic + Angular)
- MEAN Stack Developer (MongoDB, Express.js, Angular, Node.js)
- Technical Lead (Angular)
- SPA (Single Page Application) Developer
- Web Application Developer
- UI/UX Designer/Engineer
- Solutions Architect (Angular)
Questions and answers
Currently there are no Q&As for this course. Be the first to ask a question.
Reviews
Currently there are no reviews for this course. Be the first to leave a review.
Provider
Uplatz is leading global provider of IT & Technology training.
We have a strong network of qualified and experienced tutors. Uplatz provides training on cutting-edge technologies such as Data Science, Machine Learning, AWS, Microsoft Azure, Google Cloud, IBM Cloud, Data Engineering, Python, R, Java, SAP, Oracle, SAS, Salesforce, Web Development Stack, JavaScript, ReactJS, AngularJS, NodeJS, JSP & Java Servlets, MongoDB, BI Tools such as Tableau, Spotfire, Power BI, DW & ETL tools such as Informatica, IBM DataStage, Talend, DevOps, Project Management, Software Testing, and many more.
We provide training courses in both online formats - 1) live tutor-led, 2) self-paced videos
We feel proud to say that we are making learning affordable by keeping prices of all our courses very low. Our course prices are listed at almost 90% discounted rate from average market price.
Our Vision
- To become a global leader in the learning sector by providing training on job-oriented technologies
Our Mission
- To provide high-quality training on industry-demanded technologies
- To make learning affordable for the masses by keeping our prices extremely low
- To help our talented students get a high-paying job in the market
Will I get a Certificate of Course Completion?
Course Completion Certificate is awarded by Uplatz
What are your top courses?
- Data Science
- Machine Learning
- Cloud Computing - AWS, Azure, Google Cloud, IBM Cloud, and more
- Data Engineering
- SAP modules such as S/4HANA Finance, EWM, TRM, FICO, BPC, HCM, WM, MM, PP, PM, QM, SD, TRM, SuccessFactors, UI5 and Fiori, S/4HANA Logistics, TM, etc.
- Oracle
- SAS
- Salesforce
- BI Tools - Tableau, Power BI, Spotfire, MicroStrategy, etc.
- DW & ETL Tools - Informatica, Talend, IBM DataStage, etc.
- Project Management & DevOps
- Software Testing
- Digital Marketing & SEO
Will I be provided study material, tutor notes and practice assignments?
Uplatz provides tutor notes, practice assignments, practice sessions, and a lot of useful study material for free. This will help you in job interviews and certification exams.
In case of tutor-led online training, will I have access to the recorded sessions?
Yes. All live classes get recorded and life-time access on the recordings is provided.
Course Search and Enrollment
Simply search the course of your choice! We have a portfolio of more than 1000 courses with the premium ones highlighted clearly. Just search for the course that you want to take or simply use our online course finding tool to help you choose the best courses as per your needs and market demand.
Uplatz differentiates itself by providing extremely affordable learning to all and that too in the comfort of their homes.
Just contact us for a customized quote, your preferable timings, your affordability, and we'll work out the best course for you that will provide you not only a great return on investment but also to help you get a job with a premium salary. We'll also get you prepared for the certification exams.
Legal information
This course is advertised on Reed.co.uk by the Course Provider, whose terms and conditions apply. Purchases are made directly from the Course Provider, and as such, content and materials are supplied by the Course Provider directly. Reed is acting as agent and not reseller in relation to this course. Reed's only responsibility is to facilitate your payment for the course. It is your responsibility to review and agree to the Course Provider's terms and conditions and satisfy yourself as to the suitability of the course you intend to purchase. Reed will not have any responsibility for the content of the course and/or associated materials.