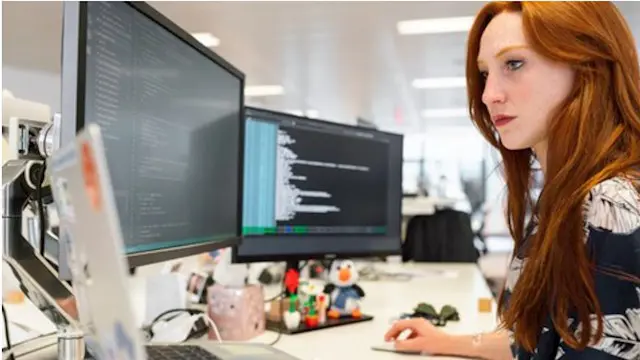
Developing a Multithreaded Kernel From Scratch!
Build a multitasking operating system and kernel with an interactive shell!
Dragon Zap
Summary
- Reed courses certificate of completion - Free
Overview
What you'll learn
How to create a kernel from scratch
How to create a multi-tasking kernel
How to handle malicious or problematic programs in your operating system. Terminating them if they misbehave.
How memory works in computers
The difference between kernel land, user land and the protection rings that make up modern computing
Kernel design patterns used by the Linux kernel its self
You will learn all about virtual memory and how to map virtual addresses to physical addresses
You will learn how to make the kernel understand processes and tasks
You will learn how to load ELF files
You will learn how to debug disassembled machine code
You will learn how to debug your kernel in an emulator with GDB.
Curriculum
Course media
Description
This course is designed to teach you how to create your very own multitasking operating system and kernel from scratch. It is assumed you have no experience in programming kernels and you are taught from the ground up.
Real Mode Development
Real mode is a legacy mode in all Intel processors that causes the processor to start in a legacy state, it performs like the old 8086 Intel processors did back in the way.
In the "Real Mode Development" section of the course we start by learning about the boot process and how memory works, we then move on to creating our very own boot loader that we test on our real machine! This boot loader will output a simple "Hello World!" message to the screen and we write this boot loader in purely assembly language.
In this section we also read a sector(512 bytes) from the hard disk and learn all about interrupts in real mode and how to create them.
This section gives you a nice taster into kernel development, without over whelming you with information. You are taught the basics and enough about the legacy processors to be able to move forward to more modern kernel development further into this course.
Protected Mode Development
In this section we create a 32 bit multi-tasking kernel that has the FAT16 filesystem. Our kernel will use Intel's built in memory protection and security mechanisms that allow us to instruct the processor to protect our kernel and prevent user programs from damaging it.
This section is very in depth, you are taught all about paging and virtual memory. We take advantage of clever instructions in Intel processors to allow all processes to share the same memory addresses, this is known as memory virtualization. We map memory addresses to point to different physical memory addresses to create the illusion that every process that is running is loaded at the same address. This is a very common technique in kernel development and is also how swap files work (Those files that are used to compensate for when you run out of usable RAM).
We create our own virtual filesystem layer that uses a design that is similar to the Linux kernel. This clever abstraction that will be taught to you was inspired by the instructors knowledge of writing Linux kernel drivers in his past.
You are taught about the design of the FAT16 filesystem and how the FAT16 filesystem is broken down into clusters and that they can chain together. We then implement our very own FAT16 filesystem driver allowing files to be born!
We implement functionality for tasks and processes and write our own keyboard drivers.
In this course you also get to learn how memory management works, we implement the "malloc" and "free" functions creating our very own heap that's designed to keep track of what memory is being used. Memory management is essential in any operating system and kernel.
Let us not forget that we even create an ELF file loader, we will compile all our operating systems programs into ELF files and allow the loading of binary programs or ELF programs. ELF files contain a lot of information that describes our program for example where our program should be loaded into memory and the different sections of the program.
By the end of this course you will have a fully functioning 32 bit multi-tasking kernel that can have many processes and tasks running at the same time. You will have a working shell that we can use as well.
Assembly language bonus
This is a bonus section designed to bring your assembly skills up to scratch should you struggle a little bit with the assembly language in this course. It's however advised you come to this course with experience in assembly language, we do use it and its important. Never the less if you want to take a chance on this course with no assembly experience then this section will help point you in the right direction so your able to take what you learned and apply it to the kernel.
Taught by an expert that that has created Linux kernel modules professionally in the work place.
Who is this course for?
- Beginner kernel developers who want to learn how to create kernels
Requirements
You must know the C programming language
It is wise have some basic knowledge in assembly language
You should have a Linux operating system, free to install from the internet (We use Ubuntu in this course)
Questions and answers
Currently there are no Q&As for this course. Be the first to ask a question.
Certificates
Reed courses certificate of completion
Digital certificate - Included
Will be downloadable when all lectures have been completed
Reviews
Currently there are no reviews for this course. Be the first to leave a review.
Legal information
This course is advertised on reed.co.uk by the Course Provider, whose terms and conditions apply. Purchases are made directly from the Course Provider, and as such, content and materials are supplied by the Course Provider directly. Reed is acting as agent and not reseller in relation to this course. Reed's only responsibility is to facilitate your payment for the course. It is your responsibility to review and agree to the Course Provider's terms and conditions and satisfy yourself as to the suitability of the course you intend to purchase. Reed will not have any responsibility for the content of the course and/or associated materials.